Table Of Content
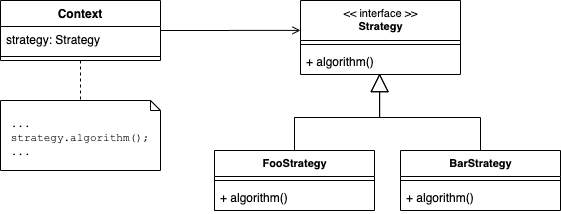
For the sake of simplicity, think about what happens when you follow someone on Twitter. You are essentially asking Twitter to send you (the observer) tweet updates of the person (the subject) you followed. The pattern consists of two actors, the observer who is interested in the updates and the subject who generates the updates. He demonstrates that 16 out of the 23 patterns in the Design Patterns book (which is primarily focused on C++) are simplified or eliminated (via direct language support) in Lisp or Dylan.

Abstract Factory Pattern
This section aims to provide examples of how we can use Async to improve the quality of our error reporting and make our code resilient. This does exactly what we need, but our add function is no longer a simple a + b. It has to first lift our values into Maybes, then reach into them to access the values, and then return the result. We need to find a way to preserve the core functionality of our add function while allowing it to work with values contained in ADTs!
Mobile App
Some alternatives could be to send back cached data, to give responses that can be chosen later, or to forward requests to several services at the same time. It helps prevent cascading failures and maintains system stability, ensuring reliable performance in distributed architectures. Exporting various types of objects into XML format via a visitor object.
Similar Reads
The Entity-Component-System - C++ Game Design Pattern (Part 1) - GameDev.net
The Entity-Component-System - C++ Game Design Pattern (Part .
Posted: Tue, 21 Nov 2017 08:00:00 GMT [source]
When the problem is identified, the most fitted design pattern can be selected. The implementation phase involves creating the necessary classes and interfaces and establishing the relationships specified by the chosen pattern. JavaScript design patterns are the reusable solutions that are applied to commonly occurring issues/problems in writing JavaScript web applications. These patterns actually help to write organized, beautiful and well-structured codes. So design patterns are one of those language-agnostic concepts you’d come across as a software engineer.

That abstract factory calls the corresponding concrete factory given the corresponding logic. Creational patterns consist of different mechanisms used to create objects. There is also another concept called Hooks.Hooks are methods in abstract classes that can be overriden, but not have to. Since slices is a required field, Builder’s private constructor is hidden and has a simple factory method that only permits to initialize with slices. While the above example is correct (and used in most examples), this is a bad practice.
Watch the Project Video
They are blueprints that can be taken and customized to solve a particular design problem in your code. Refactoring.Guru makes it easy for you to discover everything you need to know about refactoring, design patterns, SOLID principles, and other smart programming topics. What if two different clients access the Singleton class at the same time, right to the millisecond? Well, they will check if the instance is null at the same time, and will find it true, and so will create two instances of the class for each request by the two clients.
Prototype allows us to hide the complexity of making new instances from the client. The concept is to copy an existing object rather than creating a new instance from scratch, something that may include costly operations. The existing object acts as a prototype and contains the state of the object.
9 Clean Code Patterns I wish I knew earlier by Martin Thoma - Towards Data Science
9 Clean Code Patterns I wish I knew earlier by Martin Thoma.
Posted: Sat, 16 Oct 2021 07:00:00 GMT [source]
Behavioral design patterns
The specific implementation of the patterns may vary depending on many different factors. But what's important is the concepts behind them, and how they might help us achieve a better solution for our problem. While it sounds easy, as the application gets larger in the future, we may unknowingly modify the template to not be suitable for all subclasses.
The sequence on Identity is pretty dumb — it just maps over the inner type and returns the contents wrapped in an Identity container. For List and Array, sequenceuses reduce on the list to combine its contents using ap and concat. Let’s see this in action in our refactored totalLength implementation. The second method would be to use the converge combinator to achieve similar results. It then applies the input to the second and third function and pipes the results of both into the first. Let’s use it to create a function that normalizes an Arrayof objects based on their ids.
You can even write your own decorator to your program by simply extending the java.io package decorators’ superclass (FilterInputStream) and wrap it around the base class FileInputStream. Again, if one day you want to change the way how PostgreStrategy or CouchbaseStrategy works, you won’t need to touch the client code. Suppose you build a game that has a Cat class that implements a Diet class. But let’s say your cat gained too much weight and now it needs to go on a diet that will help it lose weight for its health. This CategoryDropdown file is a simple class with a constructor that initializes the category options we have available for in the dropdown.
Over time, we will definately develop the ability to recognize when and how to apply design patterns effectively in our software design and development efforts. The Gang of Four book, for instance, covers 23 classic design patterns categorized into creational, structural, and behavioral patterns. Start with creational patterns like Singleton, Factory Method, and Abstract Factory.
The mconcat and mreduce methods take a Monoid and a list of elements to work with, and apply concat to all of their elements. The only difference between them is that mconcat returns an instance of the Monoid while mreduce returns the raw value. The mconcatMap and mreduceMap helpers work in the same way, except that they accept an additional function that is used to map over every element before calling concat. We first lift our curried add function into a Maybe, and then apply Maybe aand Maybe b to it. We’ve been using map so far to access the value inside a container and ap is no different.
Use the Strategy when you have a lot of similar classes that only differ in the way they execute some behavior. Use the Strategy pattern when you want to use different variants of an algorithm within an object and be able to switch from one algorithm to another during runtime. The first version of the app could only build the routes over roads. So with the next update, you added an option to build walking routes.
They have been documented and refined over time by experienced developers and software architects. If you've ever used the MVC pattern, you've already used the observer design pattern. The Model part is like a subject and the View part is like an observer of that subject. Then you have observers, like different components, that will get that data from the subject when the data has been updated.
Design Patterns in Python introduces a powerful and essential concept in software development – design patterns. These patterns offer time-tested solutions to recurring design problems, providing a structured and efficient approach to building robust, scalable, and maintainable software systems in Python. Strategy is a behavioral design pattern that lets you define a family of algorithms, put each of them into a separate class, and make their objects interchangeable. Together, these examples showcase how different programming patterns can be employed to solve common software design challenges effectively. By understanding and applying these patterns, developers can write more maintainable, scalable, and robust software.
We’ve seen how to use Maybe and friends to ensure that we’re always working with the types we expect. But what happens when we’re working with a type that contains other values, like an Array or a List for example? Let’s look at a simple function that gives us the total length of all strings contained within an Array.
No comments:
Post a Comment